How to Use Google Map Api ( JS Script ) in Your Laravel Project
If you want to learn how to implement Google Map in Laravel , then this article is for you . In this article you will learn how to implement Google Map in Laravel using JavaScript . Your Just need to follow some basic steps in order to do this .
Table of Content :
- Get Your Google API Key
- Enable Google Map API
- Get the Map Script
- Setup your Route
- User Script in View file
Step 1 - Get Your Google API Key :
First thing we need to do is to generate our google API key for accessing google map . Visit the following link to get started .
https://cloud.google.com/maps-platform/?utm_source=google&utm_medium=cpc&utm_campaign=FY18-Q2-global-demandgen-paidsearchonnetworkhouseads-cs-maps_contactsal_saf&utm_content=text-ad-none-none-DEV_c-CRE_304562072609-ADGP_Hybrid+%7C+AW+SEM+%7C+BKWS+~+Google+Maps+API-KWID_43700037894666237-kwd-1952727095-userloc_9075215&utm_term=KW_google%20map%20api-ST_google+map+api&gclid=CjwKCAjwkdL6BRAREiwA-kiczEuzni8V2MdiW5aLu-7-Pbz3P8_FfFfOa_U-QXogfrBHHJvTsJ8pHhoCvLsQAvD_BwE
You will get an interface like the following and simply click on Get Started button .
Then on the left side corner you will see a menubar , simply click on the menubar and on the APIs and Services section click on the credentials as shown in following .
Now the next step is to create a project . simply click on the create project button present on the right side of the screen as shown following .
Now provide your project details and create your project as shown below .
Now the next step is to create a project . simply click on the create project button present on the right side of the screen as shown following .
Now provide your project details and create your project as shown below .
Now your project will be created and just click on the create credential button available on the top of the page as shown below .
After clicking on CREATE CREDENTIALS click on API Key and you will get your API Key as shown below .
Finally we got our API Key for working with Google APIs including Google Map . Copy your API code we will use it in our project .
Step 2 - Enabling Google Map API :
Now simple visit your Dashboard and click on ENABLE APIs AND SERVICES as shown below .
After that just click on " Map Javascript API " as shown below .
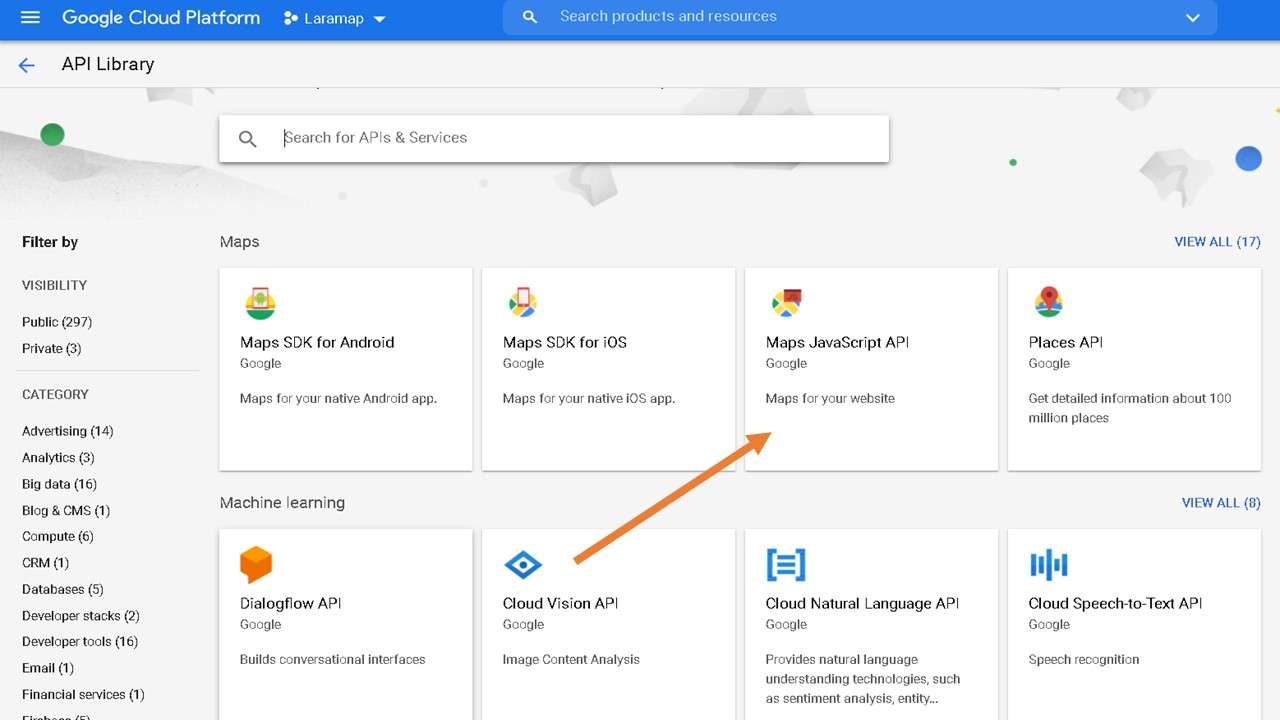
Now we are ready to implement Map API inside our Laravel Project .
or you can simply visit Google's official site to get the codes .
Step 3 - Setup Route :
Setup your route for accessing the view file .Route::get('/map',function(){ return view('map'); });
Step 4 - Get the Map Script :
map.blade.php :
@extends('layouts.app') @section('content') <div> <h1>your other page content</h1> </div> <div id="map" style="height: 350px;width: 100%;"> </div> <div> <h1>your other page content</h1> </div> @endsection @section('scripts') <script> // Initialize and add the map function initMap() { // The location of Uluru var uluru = {lat: -25.344, lng: 131.036}; // The map, centered at Uluru var map = new google.maps.Map( document.getElementById('map'), {zoom: 4, center: uluru}); // The marker, positioned at Uluru var marker = new google.maps.Marker({position: uluru, map: map}); } </script> <!--Load the API from the specified URL * The async attribute allows the browser to render the page while the API loads * The key parameter will contain your own API key (which is not needed for this tutorial) * The callback parameter executes the initMap() function --> <script defer src="https://maps.googleapis.com/maps/api/js?key=YOUR API KEY&callback=initMap"> </script> </body> </html> @endsection
or you can simply visit Google's official site to get the codes .
Note :
On the javascript section Provide your own API Key as shown below .
<script defer src="https://maps.googleapis.com/maps/api/js?key=YOUR_API_KEY&callback=initMap"> </script>
Output :
Thank you for reading this article 😊
For any query do not hesitate to comment 💬